Parameterized assessments allow instructors to author one question, with some randomized variables, which will then generate a large set of unique assessments.
Students are assigned one of the many instances of the question - often meaning they have a unique or individualized assessment.
Codio recently launched support for parameterized assessments within our existing assessment engine.
Learn more about using parameterized assessments as part of an "Evergreen Curriculum" in our webinar "Moving Beyond Detecting Cheating: Creating "Evergreen" Course Materials with Randomized & Parameterized Assessments."
Here are the basics of using parameters in Codio assessments:While the tooling is pretty simple, designing parameterized assessments can be challenging. To help get you started and understand their potential use, below are a few examples across assessment types.
Multiple Choice - What is the expected output?
One of the easiest ways to use parameters is to vary small details in questions - like loop conditions in this predict the output question:Variables x and y allow us to control the range on the outer loop, while variables a and b allow us to control the variables on the inner loop.
We then go to the PARAMETERS tab to declare these four variables:There is a handy “Add Code Example” button on the right-hand side of the screen which will import random, declare the codio_parameters dictionary and provide a sample of the syntax.
You can also use the “Generate Sample Parameters” button on the right-hand side to check your code and see an example set of parameters in the small box below the button.
The next part of this question is handling the answer choices. These need to be different depending on the variables which are being randomly selected. We can simply specify four options based on misconceptions we know students often have about nested loop execution (e.g. an off by one error, mixing up rows and columns):
Back in the PARAMETERS tab, we generate the four answers by copying the code from the question and swapping out prints for string concatenation:The PARAMETERS tab is just a python script - so the full power of the core python programming language is available to you! Need a library added? Contact support through our in-product chat or email help@codio.com.
Previewing the assessment within the assignment authoring interface makes it easy to see where variables are used in the assessment:After you publish, you can see an example of the assessment by either logging in as a Test Students or using the Preview button on the course dashboard:
To make the counting easy on the students, this assessment was set up to make rows and columns which are less than 10. This feels limiting in terms of randomization, but with the 4 variables each with 2, 3, or 4 options, we have 48 different unique instances of this question.
Fill in the Blank - Vocabulary Matching
One of our favorite Codio tricks is using a markdown table in a Fill in the Blanks question to create a matching assessment. We set it up with four vocabulary words and definitions, and three distractors using mustache templating:
This creates a nice matching assessment:
In the PARAMETERS tab is a list of tuples storing vocabulary word and definition pairs. These are shuffled using random.shuffle and the first four terms and definitions are assigned to the table variables and last three terms are assigned to the distractor variables:
After you publish, you can see an example of the assessment by either logging in as a Test Students or using the Preview button on the course dashboard:
If you consider order important, 7 choose 4 creates 840 unique matching tables. Ignoring the order of the rows, 7 choose 4 means there are 35 unique subsets of vocabulary words.
Parsons - Creating Parent and Child Classes
Parameterized assessments even work well with Parsons problems. We can use variables to randomize the names and attributes in a simple parent and child class:
The lines of code that become blocks use the same mustache templating:
When previewing we can see that the variables are used many times within the assessment:
Similar to the vocabulary exercise above, we create some nested structures and use random.shuffle to select a specific example at random. The main list indexes parent classes, the first next list is potential child classes, and the second nested list has potential class attributes:
Assuming the order of class attributes matters since it determines which class they belong to, 2 possible parent classes with 5 possible child classes, with 2 out of 8 possible class attributes results in 560 unique Parsons assessments.
Input/Output Code Tests - 2D List Manipulation
So far we have covered formative assessments, but coding exercises can be parameterized too (even using Codio’s scriptless Input/Output Code Test)!
As an example, the assessment below asks students to manipulate a 2D list:The first two cases that should be tested are (1) can they match the provided 3x3 pattern and (2) can they correctly shift their pattern. We start with these two cases and can hard-code the inputs since those are the same regardless of the pattern:
In the PARAMETERS tab, we pick a random spotted animal just for fun, but the main randomization is creating the 3x3 pattern that is constructed in the `spot` 2D list and converted to a String called `spot_pattern`:
As mentioned above, core Python is fully available in the PARAMETERS tab - meaning I can create a handy `list_to_string` helper method.
The second part of the code is generating the `spot_pattern` in the top-left corner of the 25x25 swatch (stored in `zero_zero`) for the first test case, and then generating the `spot_pattern` in the bottom right corner for the second test case.
After you publish, you can see an example of the assessment by either logging in as a Test Students or using the Preview button on the course dashboard:We provide some starter code to handle the user input for the students since the objective is 2D list manipulation, and the unique 3x3 spot pattern has been generated and is printed on the right as part of the question specification.
The randomness of the pattern requires students to figure out the unique row and column math for their specific pattern – one of 126 possible patterns.
Learn more about using parameterized assessments as part of an "Evergreen Curriculum" in our webinar "Moving Beyond Detecting Cheating: Creating "Evergreen" Course Materials with Randomized & Parameterized Assessments."
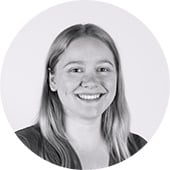